Functions:
A function is a group of statements that together perform a task.
Function takes inputs, do some operations and produces output.
Every C program has at least one function, which is nothing but main() functions.
Syntax:
Return_type function_name( parameter list )
{
Body of the function
}
i. Return type: A function mat return a value. The return_type is the data type of the value the function returns.
ii. Function Name: This is the actual name of the function.
iii. Parameters: A parameter is like a placeholder. When a function is invoked, then we can pass the value to the parameter. This value is referred to the actual parameter or argument.
iv. Function body: The function body contains a collections of statements that define a function does.
Functions are classified into two types
1. System defined functions
2. User defined functions
1. System defined functions:
These functions are already defined by the compiler. example printf(),scanf(),clrscr() etc.
2. User defined functions:
These functions are defined by the user at the time of writing a program
EX: Function definition
int addition(int first_number, int second_number);
Function Calling:
While creating a c function, we define a function of what has to do. To use the defined function we have to call the function to perform the defined task.
Input/output functions:
Input: Input is nothing but to provide some data to be used in the program.
Output: Output is nothing but to display data on the screen.\
C language provides many built in functions to read and display the data.
i. Printf(): It is used to print something on output screen.
Syntax: printf("format specifiers", variable1, variable2);
ii. Scanf(): It is used to accept values from user.
Syntax: scanf("format specifiers", &variable1, &variable2);
iii. Getchar(): This function reads a character form the terminal and returns as an integer.This function reads single character at a time.
iv. Putchar(): This function displays a character passed to it on the screen.This function reads single character at a time.
v. Puts: This function displays the string.
vi: Gets:This function reads a line into the buffer until new line or end of file occurs.
Ex:

Output:

Ex:

A function is a group of statements that together perform a task.
Function takes inputs, do some operations and produces output.
Every C program has at least one function, which is nothing but main() functions.
Syntax:
Return_type function_name( parameter list )
{
Body of the function
}
i. Return type: A function mat return a value. The return_type is the data type of the value the function returns.
ii. Function Name: This is the actual name of the function.
iii. Parameters: A parameter is like a placeholder. When a function is invoked, then we can pass the value to the parameter. This value is referred to the actual parameter or argument.
iv. Function body: The function body contains a collections of statements that define a function does.
Functions are classified into two types
1. System defined functions
2. User defined functions
1. System defined functions:
These functions are already defined by the compiler. example printf(),scanf(),clrscr() etc.
2. User defined functions:
These functions are defined by the user at the time of writing a program
Function Declaration:
A function declaration tells the compiler about a function name and how to call the function. The actual body of the function can be defined separately.EX: Function definition
int addition(int first_number, int second_number);
Function Calling:
While creating a c function, we define a function of what has to do. To use the defined function we have to call the function to perform the defined task.
Input/output functions:
Input: Input is nothing but to provide some data to be used in the program.
Output: Output is nothing but to display data on the screen.\
C language provides many built in functions to read and display the data.
i. Printf(): It is used to print something on output screen.
Syntax: printf("format specifiers", variable1, variable2);
ii. Scanf(): It is used to accept values from user.
Syntax: scanf("format specifiers", &variable1, &variable2);
iii. Getchar(): This function reads a character form the terminal and returns as an integer.This function reads single character at a time.
iv. Putchar(): This function displays a character passed to it on the screen.This function reads single character at a time.
v. Puts: This function displays the string.
vi: Gets:This function reads a line into the buffer until new line or end of file occurs.
Ex:
Output:
Ex:
Output:

Mathematical Functions:
i. Ceil(): This function returns the smallest integer value greater than or equal to specified value.
ii. Floor(): This function returns the largest integer value greater than or equal to specified value.
iii. Abs(): It returns the absolute value of specified value.
iv: Sqrt(): It returns the square root of specified value.
v.Pow: It returns the power of first value against second value.


File input and output functions:
A file represents a sequence of bytes, regardless of it being a text file or binary file.
1. fopen(): Open an existing file and creation of a bew file with attributes as "a","a+","w","w++"
2. fscanf(): Reads a set of data from file.
3. fprintf(): Writs a set of data to a file.
4. fseek(): Set the position to desire point in a file.
5. rewind(): Set the position to the beginng point.
6. fclose(): Closing a file
7. getc(): Reads a character from a file.
8. putc(): Writes a character to a file.
9. getw(): Reads a integer from a file.
10. putw(): Writes a integer to a file.
11.ftell(): Gives the current position in the file.
Task-1: Addition of 2 Numbers using functions

Output:

Task-2: Multiplication table using functions

Output:

String:
A string is an array of characters terminated with a null character.
When compiler encounters a sequence of characters enclosed in double quotation marks, it appends a null character '\0' at the end.
Declaration and Initialization:
datatype array_name[array_size];
Ex: char Names[15]="SithaGeetha"
Ex-1:

Output:

Here scanf terminates second string
Ex-2:Using gets and puts

Output:

By using puts and gets function, gets function considers the space as a character and it reads the entire line
Strlen(): Strlen function is used to find the length of the string.
Ex-3: String Length
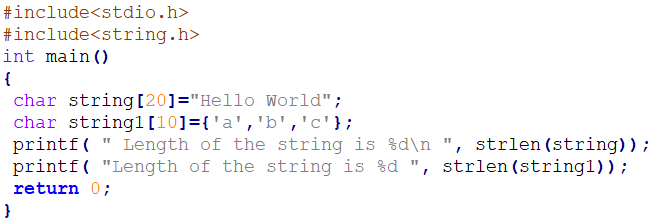
Output:

Strcpy(): Strcpy function is used to copy one string into the another string.
Ex-4:String copy

Output:

Strcmp(): Strcmp function is used to compare one string with the another string
It returns '0' if both the strings are matched.
It returns '1' if the ascii value of first unmatched character is less than second.
It returns '1' if the ascii value of first unmatched character is greater than second.
Ex-5:String Comparison

Output:

Ex-6: Finding length and comparing String with Functions

Output:

Mathematical Functions:
i. Ceil(): This function returns the smallest integer value greater than or equal to specified value.
ii. Floor(): This function returns the largest integer value greater than or equal to specified value.
iii. Abs(): It returns the absolute value of specified value.
iv: Sqrt(): It returns the square root of specified value.
v.Pow: It returns the power of first value against second value.
File input and output functions:
A file represents a sequence of bytes, regardless of it being a text file or binary file.
1. fopen(): Open an existing file and creation of a bew file with attributes as "a","a+","w","w++"
2. fscanf(): Reads a set of data from file.
3. fprintf(): Writs a set of data to a file.
4. fseek(): Set the position to desire point in a file.
5. rewind(): Set the position to the beginng point.
6. fclose(): Closing a file
7. getc(): Reads a character from a file.
8. putc(): Writes a character to a file.
9. getw(): Reads a integer from a file.
10. putw(): Writes a integer to a file.
11.ftell(): Gives the current position in the file.
Task-1: Addition of 2 Numbers using functions
Output:
Task-2: Multiplication table using functions
Output:
String:
A string is an array of characters terminated with a null character.
When compiler encounters a sequence of characters enclosed in double quotation marks, it appends a null character '\0' at the end.
Declaration and Initialization:
datatype array_name[array_size];
Ex: char Names[15]="SithaGeetha"
Ex-1:
Output:
Here scanf terminates second string
Ex-2:Using gets and puts
Output:
By using puts and gets function, gets function considers the space as a character and it reads the entire line
Strlen(): Strlen function is used to find the length of the string.
Ex-3: String Length
Output:
Strcpy(): Strcpy function is used to copy one string into the another string.
Ex-4:String copy
Output:
Strcmp(): Strcmp function is used to compare one string with the another string
It returns '0' if both the strings are matched.
It returns '1' if the ascii value of first unmatched character is less than second.
It returns '1' if the ascii value of first unmatched character is greater than second.
Ex-5:String Comparison
Output:
Ex-6: Finding length and comparing String with Functions
Output: