Identifiers:
Identifiers are the names given to variables,functions etc..
Literals:
Literals are used to represent values.These values are fixed.
Literals are different types.
EX:
Code:

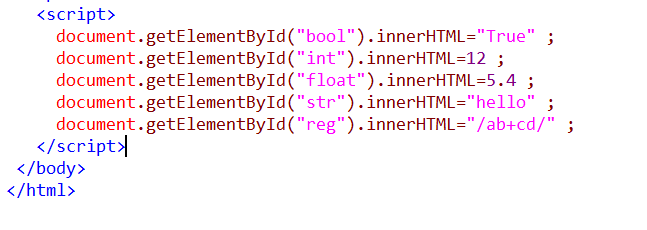
Output:

Variables:
Identifiers are the names given to variables,functions etc..
- Identifiers are case sensitive.
- keywords are not used as identifiers.
- The first character should not be a number.
- The first keyword can be an alphabet,underscore or dollar.
- No special characters are allowed except dollar and underscore.
- variables are used to store the data values.
- javascript uses the var keyword to declare variables.
- Rules to declare variables.
Literals:
Literals are used to represent values.These values are fixed.
Literals are different types.
1. Array Literal: It is a list of zero or more expressions(elements)
Ex: var=[1,2];
2. Boolean Literal:It has two literal value.True or False.
3. Integer Literals:Integers can be expressed in decimal.hexadecimal and binary
Ex:var a=42;
4. Floating point literals: fractional part of a number is known as floating point.
Ex: var b=5.1:
5. Object Literal: An object is a list of zero or more pairs of property names values associated with it.
6. RegExp: Literal is a pattern enclosed in slaches.
Ex:var re= \ab+c\;
String Literal:Zero or more expressions enclosed in angular braces
Ex:var re= \ab+c\;
String Literal:Zero or more expressions enclosed in angular braces
EX:
Code:
Output:
Variables:
- Variables are used to store the values.
- variables values can be changed.
- Variables can not be keywords.
- These are case sensitive.
- Syntax: var var-name:value.
EX:
Code:

Output:

Expressions:
Code:
Output:
Expressions:
- Expressions are units of code that can be evaluated and resolve to a value.
- Any expression is any valid set of literals,variables and expressions that evaluates a single value.
- Syntax: var name=value or value=operand;
Code:
OUTPUT:
Comments:
- Comments are used to make the program much more easier to undersand.
- Any text between the comments will not be executed.
- In Javascript we have 2 types of comments. Single line comment
Multi line comment
1. Single line comments:
- These are represented with // comments.
- These are used for single line of statements.
- Any code between these slashes will never execute.
2. Multi line comments:
- These are Represented with /* and */.
- These are used for block of statements or group of statements.
EX:
Code:
OUTPUT:
JavaScript and Camel case:
- Javascript is case sensitive.
- The first character should not be a number.
- we can use both upper case and lower case letters.
EX: var Num1;
var num1;